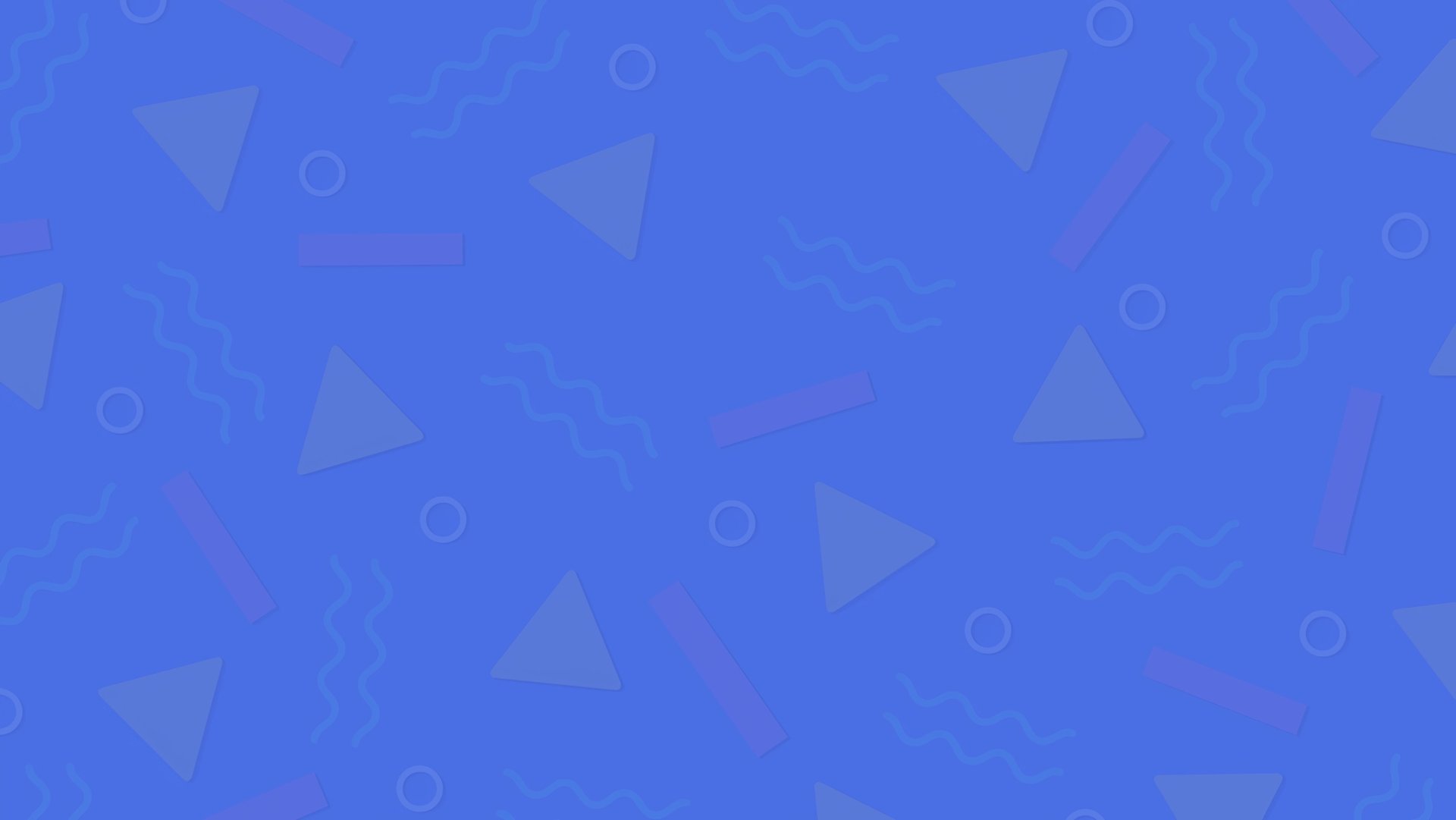
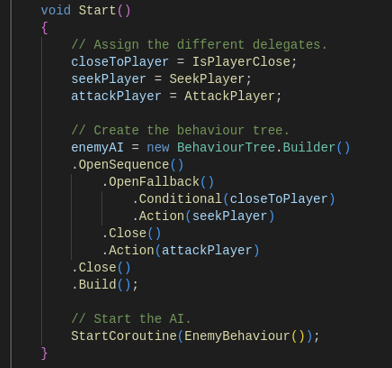
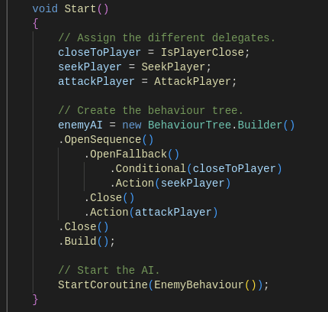
Behaviour Trees in Unity3D
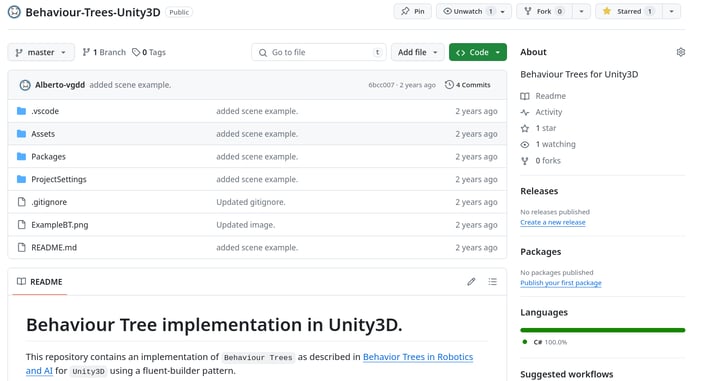
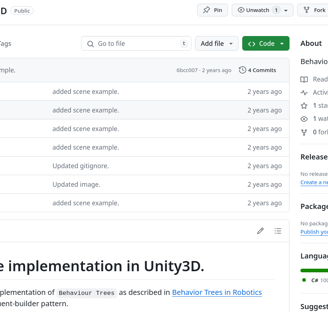
When I began programming games, I always had encountered an issue that had prevented me from exploring new ideas and genres: enemy behaviours, as I always limited myself to simplified patrolling AIs (Like the turtles from Super Mario Bros). I did some experiments using Finite State Machines, but I ended up quickly noticing its limitations: poor scalability, maintainability, and flexibility.
In consequence of that, I decided to research alternative automation patterns and I found a very interesting paper that covered the basics of Behaviour Trees and how they relate to some other alternatives: Behavior Trees in Robotics and AI: An Introduction
Complex Enemy Behaviours
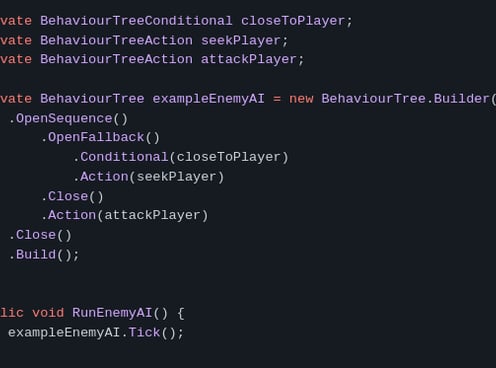
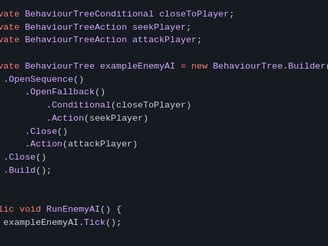
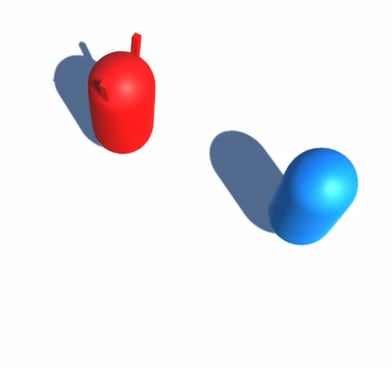
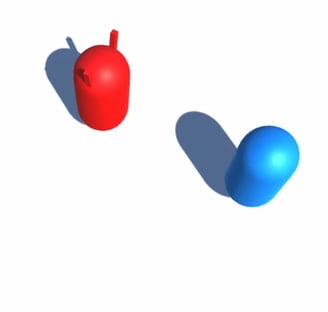
Implementing BTs
I began writing my own implementation in C# using the fluent builder pattern I have grown very used to after reading Effective Java. This implementation covers only the core components of a behaviour tree: Fallback, Sequence and Parallel control flow nodes, as well as Action and Conditional execution nodes. Furthermore, I have also included an AbstractControlFlowNode class for anyone to implement custom Decorator nodes.
I have created a GitHub that contains the codebase and a brief example behaviour tree that describes an enemy that seeks the player character and then attacks him.
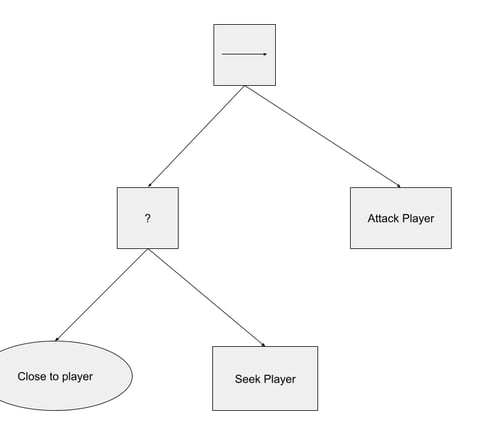
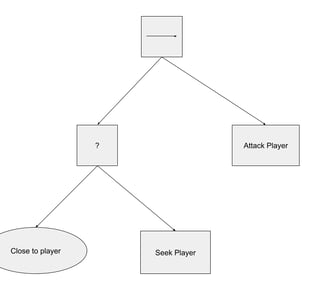
Take a look at the code here
A simple showcase
The repository also includes a sample scene that features two entities. The first one, in blue, responds to player inputs and can move around freely. The other one, in red, is controlled by an AI that uses a tree that implements the example described in the documentation for the developers to understand how to use this resource.
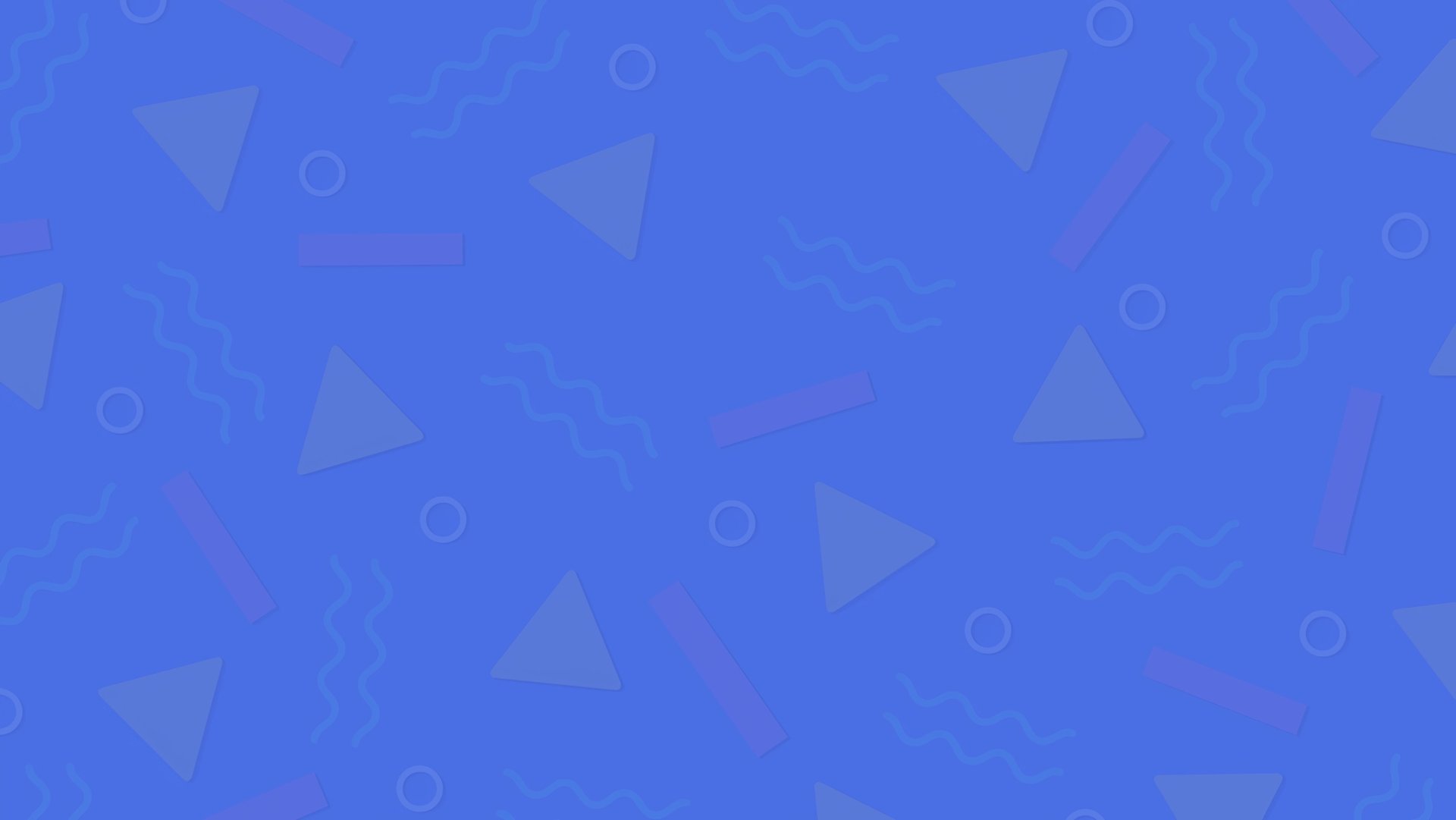